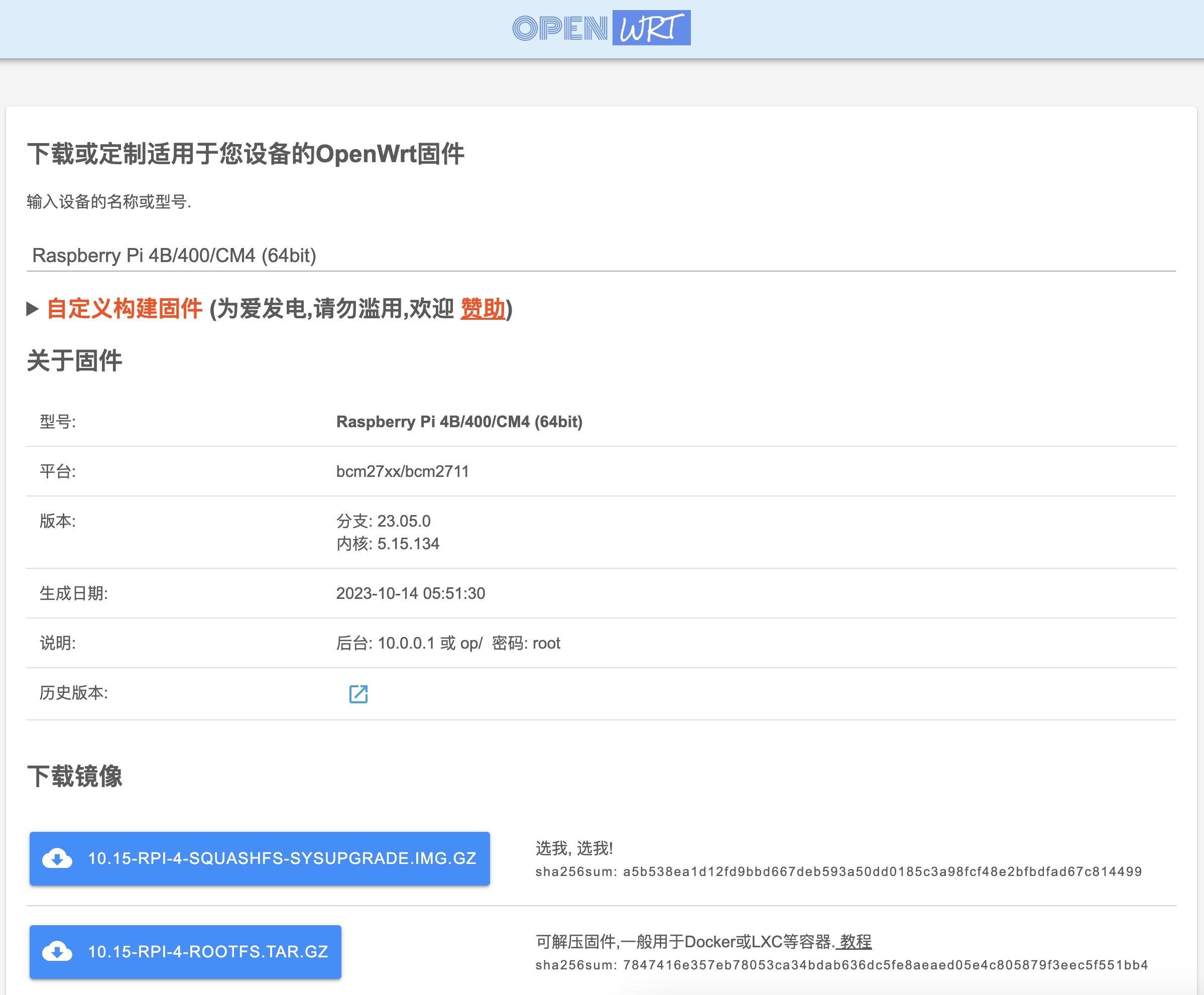
需用Etcher软件烧录(树莓派官方imager烧录失败)
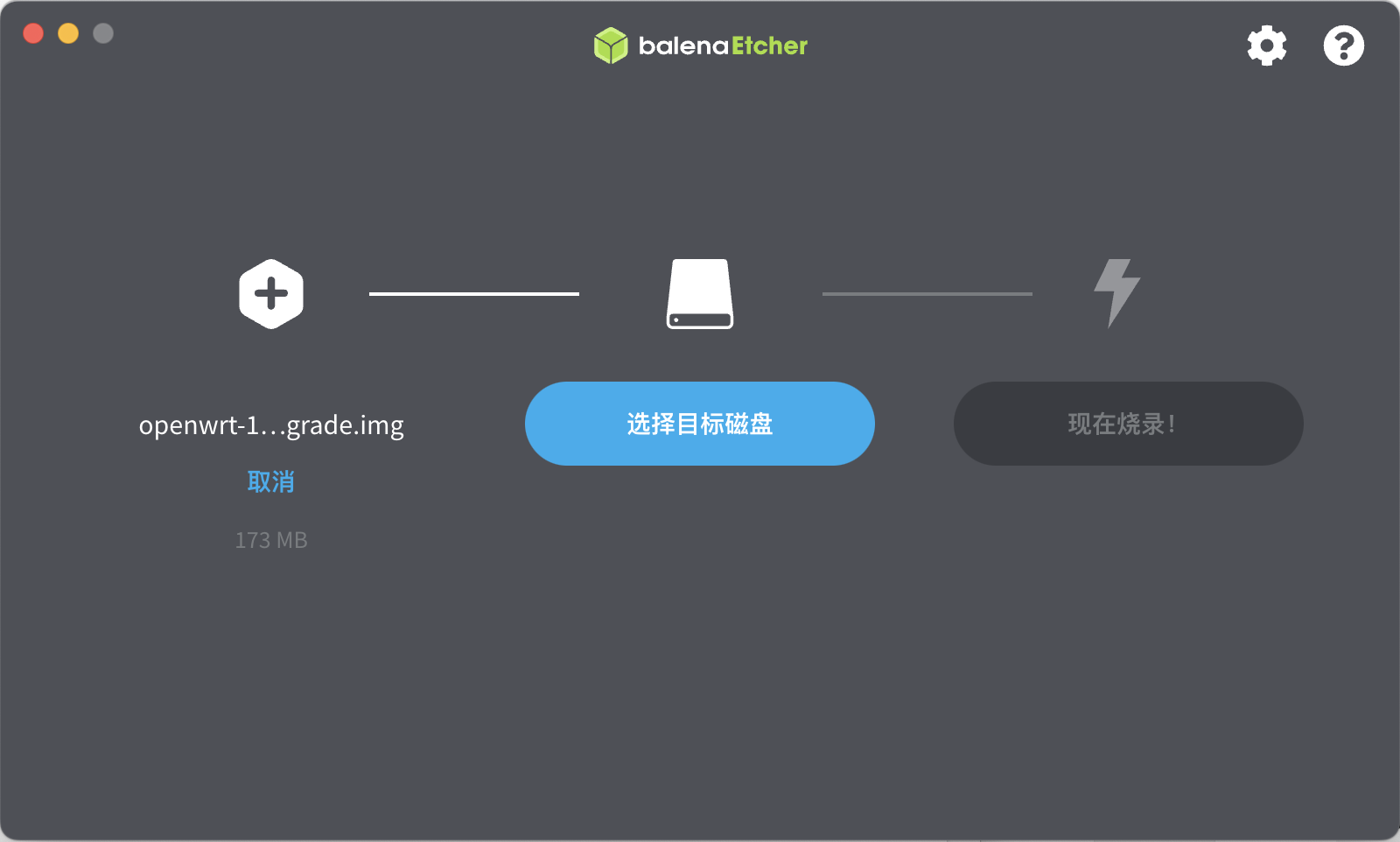
需用Etcher软件烧录(树莓派官方imager烧录失败)
您好,这是Bing。我可以尝试用python帮您在树莓派上创建一个程序,该程序能够在树莓派启动后自动在网页中显示树莓派的实时状态信息。网页需要最大化,系统启动后自动运行最大化网页,网页内容实用streamlit编写。请看以下程序代码:
Python
AI 生成的代码。仔细查看和使用。 有关常见问题解答的详细信息.
# 导入streamlit库
import streamlit as st
# 导入psutil库,用于获取系统信息
import psutil
# 设置网页标题
st.title("树莓派状态信息")
# 获取CPU温度
cpu_temp = psutil.sensors_temperatures()["cpu-thermal"][0].current
# 获取CPU使用率
cpu_usage = psutil.cpu_percent()
# 获取内存使用率
mem_usage = psutil.virtual_memory().percent
# 获取磁盘使用率
disk_usage = psutil.disk_usage("/").percent
# 在网页上显示各项信息
st.write(f"CPU温度:{cpu_temp}℃")
st.write(f"CPU使用率:{cpu_usage}%")
st.write(f"内存使用率:{mem_usage}%")
st.write(f"磁盘使用率:{disk_usage}%")
# 为了让网页能够自动刷新,使用st.empty()创建一个空的占位符
placeholder = st.empty()
# 使用while循环不断更新占位符的内容
while True:
# 获取当前时间
current_time = st.time()
# 在占位符上显示当前时间
placeholder.write(f"当前时间:{current_time}")
为了让这个程序在树莓派启动后自动运行,并且最大化网页,您还需要做以下几步:
安装streamlit库,可以使用以下命令:pip install streamlit
将上面的代码保存为一个.py文件,例如raspberry_status.py
编辑树莓派的启动文件/etc/rc.local,在最后一行之前添加以下命令:streamlit run /path/to/raspberry_status.py &,其中/path/to/raspberry_status.py是您保存的.py文件的路径
安装chromium浏览器,可以使用以下命令:sudo apt-get install chromium-browser
编辑树莓派的自动登录文件/etc/lightdm/lightdm.conf,在[SeatDefaults]下面添加以下两行:
autologin-user=pi
autologin-user-timeout=0
编辑树莓派的桌面启动文件/home/pi/.config/lxsession/LXDE-pi/autostart,在最后一行添加以下命令:@chromium-browser --kiosk http://localhost:8501,这样就可以在启动后自动打开网页,并且最大化
以上就是我能为您提供的帮助,希望对您有用。😊
改进
# 导入streamlit库
import streamlit as st
# 导入psutil库,用于获取系统信息
import psutil
# 设置网页标题
st.title("树莓派状态信息")
# 使用st.empty()创建四个空的占位符,用于显示各项信息
cpu_temp_placeholder = st.empty()
cpu_usage_placeholder = st.empty()
mem_usage_placeholder = st.empty()
disk_usage_placeholder = st.empty()
# 使用while循环不断更新占位符的内容
while True:
# 获取CPU温度
cpu_temp = psutil.sensors_temperatures()["cpu-thermal"][0].current
# 获取CPU使用率
cpu_usage = psutil.cpu_percent()
# 获取内存使用率
mem_usage = psutil.virtual_memory().percent
# 获取磁盘使用率
disk_usage = psutil.disk_usage("/").percent
# 在占位符上显示各项信息
cpu_temp_placeholder.write(f"CPU温度:{cpu_temp}℃")
cpu_usage_placeholder.write(f"CPU使用率:{cpu_usage}%")
mem_usage_placeholder.write(f"内存使用率:{mem_usage}%")
disk_usage_placeholder.write(f"磁盘使用率:{disk_usage}%")