esp32-c3系列因其低廉的价格颇具竞争力,某宝买了个9.9的esp32-c3开发板,用micropython开发uart通信,一开始接上电路板丝印的RX、TX引脚,但一直未能通信甚至干扰了调试,咨询厂家,因C3系列较新,厂家FAE也不熟悉micropython,翻看micropython官网等也找不到相关资料,给调试工作带来很大麻烦。这里提供一个简单的方法找出uart引脚,也就一行代码:
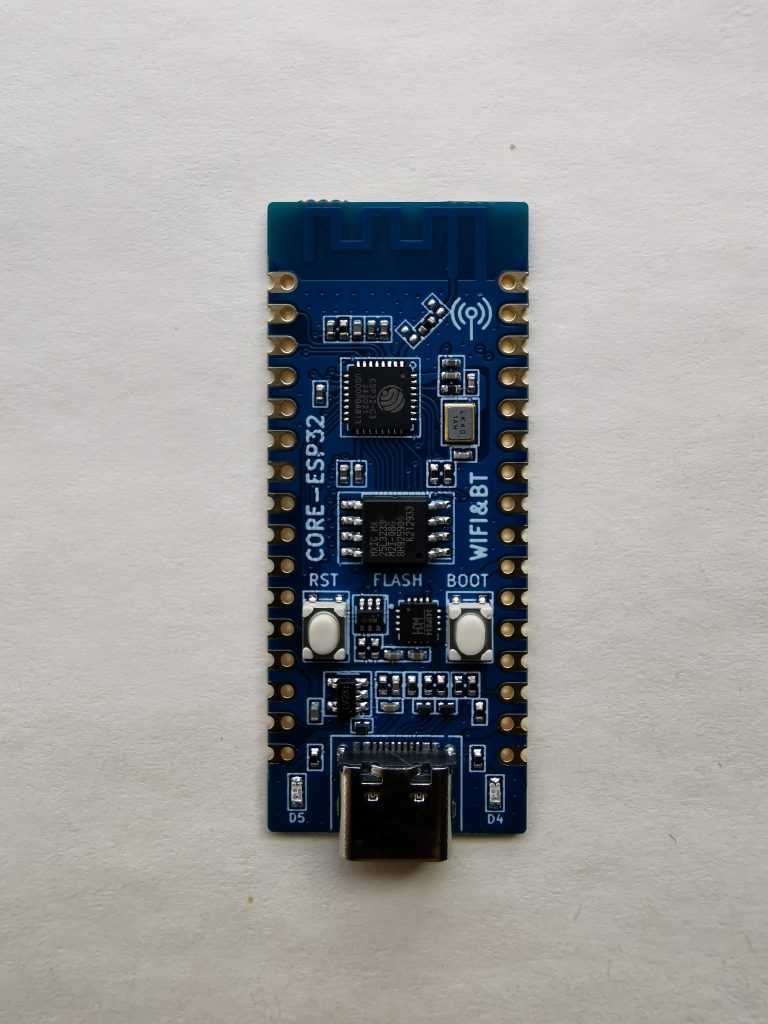
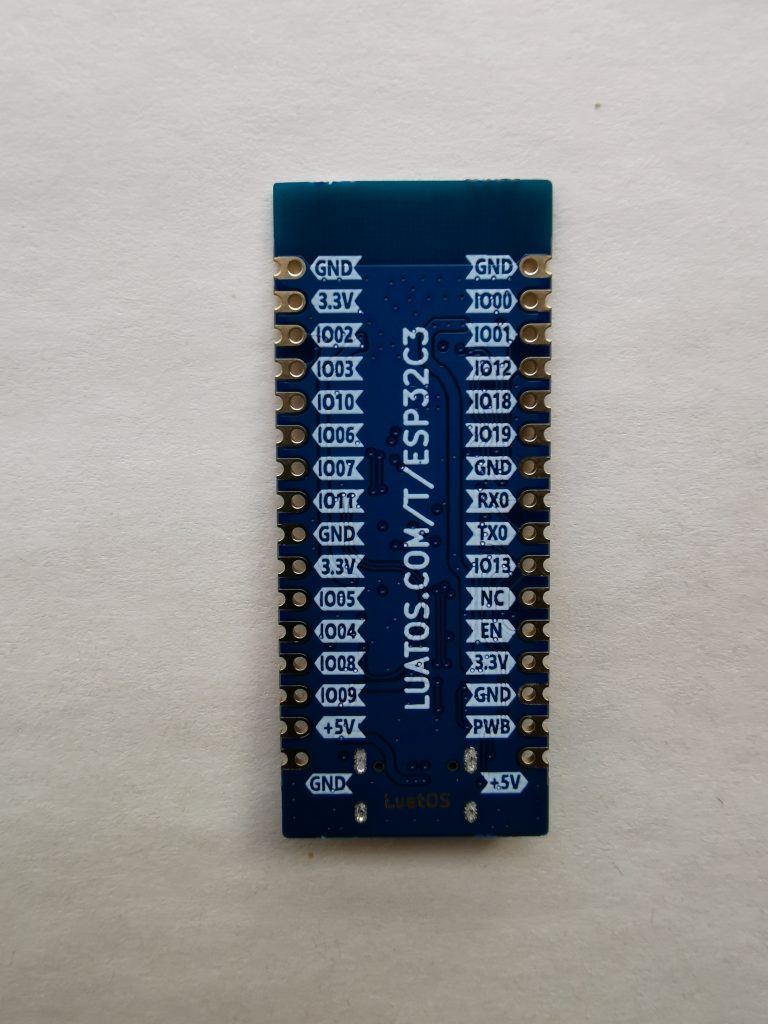
注意点一:
uart = machine.UART(1, baudrate=115200,timeout=10)
print(uart)
#此时可以看到rx=10,tx=9,但如果将树莓派的tx、rx分别接esp32C3的引脚10和9,则不会有任何信息,这是最大的坑,此时需将上述代码更新为
uart = machine.UART(1, baudrate=115200,rx=10,tx=9,timeout=10)
这样就没有任何问题了
注意点二:
上位机程序的timeout数值一定要大于等于下位机time.sleep的时间
全部代码如下:
import ntptime
import network,time
import machine
from machine import Pin,RTC
rtc = RTC()
#print("同步前本地时间:%s" %str(time.localtime()))
led=Pin(2,Pin.OUT)
# 联WIFI
def WIFI_Connect():
wlan = network.WLAN(network.STA_IF) #STA模式
wlan.active(True) #激活接口
start_time=time.time() #记录时间做超时判断
if not wlan.isconnected():
print('connecting to network...')
wlan.connect('HUAWEI', 'china') #输入WIFI账号密码
while not wlan.isconnected():
if time.time()-start_time > 15 :
print('WIFI Connected Timeout!')
break
if wlan.isconnected():
print('connected!')
print('network information:', wlan.ifconfig())
# 同步时间
def sync_ntp():
ntptime.NTP_DELTA = 3155644800 # 可选 UTC+8偏移时间(秒),不设置就是UTC0
ntptime.host = 'ntp1.aliyun.com' # 可选,ntp服务器,默认是"pool.ntp.org"
try:
ntptime.settime() # 修改设备时间,到这就已经设置好了
except:
for i in range(6):
led.value(1) #turn off 0是亮
time.sleep(0.1)
led.value(0)
time.sleep(0.1)
print('同步失败')
uart = machine.UART(1, baudrate=115200,rx=10,tx=9,timeout=10)
#uart = machine.UART(1, baudrate=115200, timeout=10)
p22 = Pin(6, Pin.OUT)
readmsg = ''
sendmsg = '1'
WIFI_Connect()
while True:
sync_ntp()
dt=time.localtime()
print(uart)
time.sleep(20)
if uart.any() > 0:
readmsg = uart.read()
print(readmsg)
if '2' in readmsg:
uart.write(sendmsg)
if dt[3]>=20:
print('power off')
p22.on()
time.sleep(0.5)
p22.off()
else:
print(readmsg)
print('reset now')
p22.on()
time.sleep(0.5)
p22.off()
elif uart.any()==0: #多次读取,防止有信息丢失导致误判
print(dt[3])
if dt[3]>=0 and dt[3]<20: #如白天读不到信息则开机
print('power on')
p22.on()
time.sleep(0.5)
p22.off()
改进版本(自动根据上位机发送的串口信息生成包含wifi及开关机时间等信息的配置文件,避免了在烧录程序时写死wifi密码等信息)
import ntptime
import network,time
import machine
from machine import Pin,RTC
uart = machine.UART(1, baudrate=115200,rx=10,tx=9,timeout=10)
readmsg = ''
sendmsg = 'huanghe'
rtc = RTC()
#print("同步前本地时间:%s" %str(time.localtime()))
led=Pin(2,Pin.OUT)
# 联WIFI
def WIFI_Connect():
wlan = network.WLAN(network.STA_IF) #STA模式
wlan.active(True) #激活接口
start_time=time.time() #记录时间做超时判断
if not wlan.isconnected():
print('connecting to network...'+ssid[1])
wlan.connect(ssid[1], pwd[1]) #输入WIFI账号密码
while not wlan.isconnected():
if time.time()-start_time > 15 :
print('WIFI Connected Timeout!')
break
if wlan.isconnected():
print('connected!')
print('network information:', wlan.ifconfig())
# 同步时间
def sync_ntp():
ntptime.NTP_DELTA = 3155644800 # 可选 UTC+8偏移时间(秒),不设置就是UTC0
ntptime.host = 'ntp1.aliyun.com' # 可选,ntp服务器,默认是"pool.ntp.org"
try:
ntptime.settime() # 修改设备时间,到这就已经设置好了
except:
for i in range(6):
led.value(1) #turn off 0是亮
time.sleep(0.1)
led.value(0)
time.sleep(0.1)
print('同步失败')
#uart = machine.UART(1, baudrate=115200, timeout=10)
p22 = Pin(6, Pin.OUT)
try:
with open('config.txt',mode='r',encoding='utf-8') as f:
s=f.read().split(',')
ssid=s[0].split(':')
pwd=s[1].split(':')
startt=s[2].split(':')
endt=s[3].split(':')
print(startt[1])
print(endt[1])
except:
print('config file error')
time.sleep(5) #必不可少,并且时间不能小于上位机发送定时
if uart.any() > 0:
readmsg = uart.read()
print(readmsg)
if 'SSID' in readmsg:
content = readmsg
with open('config.txt',mode='w',encoding='utf-8') as f:
f.write(content)
machine.reset()
try:
WIFI_Connect()
except:
print('wifi connect error')
while True:
sync_ntp()
dt=time.localtime()
print(uart)
time.sleep(20)
if uart.any() > 0:
readmsg = uart.read()
print(readmsg)
if 'changjiang' in readmsg:
uart.write(sendmsg)
print('dt[3]='+str(dt[3]))
print('endt[1]='+endt[1])
if dt[3]>=int(endt[1]):
print('power off')
p22.on()
time.sleep(0.5)
p22.off()
else:
print(readmsg)
print('reset now')
p22.on()
time.sleep(0.5)
p22.off()
elif uart.any()==0: #多次读取,防止有信息丢失导致误判
print(dt[3])
if dt[3]>=int(startt[1]) and dt[3]<int(endt[1]): #如白天读不到信息则开机
print('power on')
p22.on()
time.sleep(0.5)
p22.off()
去错版本
#coding:utf-8
import ntptime
import network,time
import machine
import os
from machine import Pin,RTC
uart = machine.UART(1, baudrate=115200,rx=10,tx=9,timeout=10)
readmsg = ''
sendmsg = 'huanghe'
startt=6
endt=20
rtc = RTC()
#print("同步前本地时间:%s" %str(time.localtime()))
led=Pin(2,Pin.OUT)
# 联WIFI
def WIFI_Connect():
wlan = network.WLAN(network.STA_IF) #STA模式
wlan.active(True) #激活接口
start_time=time.time() #记录时间做超时判断
if not wlan.isconnected():
print('connecting to network...'+ssid[1])
wlan.connect(ssid[1], pwd[1]) #输入WIFI账号密码
while not wlan.isconnected():
if time.time()-start_time > 15 :
print('WIFI Connected Timeout!')
break
if wlan.isconnected():
print('connected!')
print('network information:', wlan.ifconfig())
# 同步时间
def sync_ntp():
ntptime.NTP_DELTA = 3155644800 # 可选 UTC+8偏移时间(秒),不设置就是UTC0
ntptime.host = 'ntp1.aliyun.com' # 可选,ntp服务器,默认是"pool.ntp.org"
try:
ntptime.settime() # 修改设备时间,到这就已经设置好了
except:
for i in range(6):
led.value(1) #turn off 0是亮
time.sleep(0.1)
led.value(0)
time.sleep(0.1)
print('同步失败')
#uart = machine.UART(1, baudrate=115200, timeout=10)
p22 = Pin(6, Pin.OUT)
try:
with open('config.txt',mode='r',encoding='utf-8') as f:
s=f.read().split(',')
if 'SSID' and 'PWD' and 'START' and 'END' and 'changjiang' in s:
ssid=s[0].split(':')
pwd=s[1].split(':')
startt=s[2].split(':')
endt=s[3].split(':')
startta=startt[1]
endta=endt[1]
print(startta)
print(endta)
else:
print('file format error,will drop it')
os.remove('config.txt')
except:
print('config file not find')
startta=6
endta=20
time.sleep(5) #必不可少,并且时间不能小于上位机发送定时
if uart.any() > 26: #接受字符串个数
readmsg = uart.read()
print(readmsg)
if 'SSID' and 'PWD' and 'START' and 'END' and 'changjiang' in str(readmsg):
content = readmsg
with open('config.txt',mode='w',encoding='utf-8') as f:
# f.seek(0)
f.write(content)
machine.reset()
else:
try:
os.remove('config.txt')
except:
print('未找到待删除文件')
try:
WIFI_Connect()
except:
print('wifi connect error')
while True:
sync_ntp()
dt=time.localtime()
readmsg = uart.read()
print(uart)
time.sleep(20)
if uart.any() > 0:
readmsg = uart.read()
print(readmsg)
if 'changjiang' in readmsg:
uart.write(sendmsg)
print('dt[3]='+str(dt[3]))
print('endt[1]='+str(endta))
if dt[3]>=int(endta):
print('power off')
p22.on()
time.sleep(0.5)
p22.off()
else:
print(readmsg)
print('reset now')
p22.on()
time.sleep(0.5)
p22.off()
elif uart.any()==0: #多次读取,防止有信息丢失导致误判
print(dt[3])
if dt[3]>=int(startta) and dt[3]<int(endta): #如白天读不到信息则开机
print('power on')
p22.on()
time.sleep(0.5)
p22.off()
对应上位机程序
import serial #导入模块
with open('/home/pi/config.txt',mode='r',encoding='utf-8') as f:
s=f.read()
#端口,GNU/Linux上的/dev/ttyUSB0 等或Windows上的 COM3 等
portx="/dev/ttyAMA0"
#波特率,标准值之一:50,75,110,134,150,200,300,600,1200,1800,2400,4800,9600,19200,38400,57600,115200
bps=115200
#超时设置,None:永远等待操作,0为立即返回请求结果,其他值为等待超时时间(单位为秒)
timex=5
# 打开串口,并得到串口对象
ser=serial.Serial(portx,bps,timeout=timex)
while True:
# 写数据
send=s
result=ser.write(send.encode())
print("写总字节数:",result)
str1 =ser.readline().decode()
if('huanghe' in str1):
print(str1)
ser.close()#关闭串口
更新版本
ESP32-C3侧
#coding:utf-8
from machine import UART,Pin,RTC
import machine
import time,network
import ntptime
led=Pin(2,Pin.OUT)
rtc = RTC()
def linedetect():
uart=UART(1,baudrate=115200,tx=6,rx=7,timeout=5)
idsend='huanghe'
idreceive='changjiang'
uart.write(idsend)
time.sleep(15) #树莓派启动需要的时间,否则会反复重启
receive_data=uart.readline()
if (idreceive in str(receive_data)):
return 1
else:
return 0
def onoff(pinnum):
p = Pin(pinnum, Pin.OUT)
p.on()
time.sleep(0.5)
p.off()
def createconfigfile(filename):
uart=UART(1,baudrate=115200,tx=6,rx=7,timeout=5)
time.sleep(5)
readmsg = uart.readline()
if 'SSID' and 'PWD' and 'START' and 'END' and 'changjiang' in str(readmsg):
content = readmsg
with open(filename,mode='w',encoding='utf-8') as f:
f.write(content)
def readconfigfile(filename):
with open(filename,mode='r',encoding='utf-8') as f:
s=f.read().split(',')
if 'SSID' and 'PWD' and 'START' and 'END' and 'changjiang' in s:
ssid=s[0].split(':')[1]
pwd=s[1].split(':')[1]
startt=s[2].split(':')[1]
endt=s[3].split(':')[1]
return ssid,pwd,startt,endt
else:
print('file format error,will drop it')
os.remove(filename)
# 同步时间
def sync_ntp():
ntptime.NTP_DELTA = 3155644800 # 可选 UTC+8偏移时间(秒),不设置就是UTC0
ntptime.host = 'ntp1.aliyun.com' # 可选,ntp服务器,默认是"pool.ntp.org"
try:
ntptime.settime() # 修改设备时间,到这就已经设置好了
except:
for i in range(6):
led.value(1) #turn off 0是亮
time.sleep(0.1)
led.value(0)
time.sleep(0.1)
print('同步失败')
# 联WIFI
def WIFI_Connect(ssid,pwd):
wlan = network.WLAN(network.STA_IF) #STA模式
wlan.active(True) #激活接口
start_time=time.time() #记录时间做超时判断
if not wlan.isconnected():
print('connecting to network...'+ssid)
wlan.connect(ssid, pwd) #输入WIFI账号密码
while not wlan.isconnected():
if time.time()-start_time > 15 :
print('WIFI Connected Timeout!')
break
if wlan.isconnected():
print('connected!')
try:
a,b,c,d=readconfigfile('config.txt')
except:
print('error')
createconfigfile('config.txt')
machine.reset()
WIFI_Connect(a,b)
sync_ntp()
print(a,b,c,d)
while True:
dt=time.localtime()
print(dt)
linestate=linedetect()
if linestate!=0:
print('on line')
if dt[3]>int(d):
onoff(4)
else:
print('off line')
if (dt[3]<=int(d) and dt[3]>int(c)):
onoff(4)
'''
while True:
a=linedetect()
if a==0:
print('off line')
else:
print('on line')
'''
树莓派侧
import serial
i=0
with open('/home/pi/config.txt',mode='r',encoding='utf-8') as f:
s=f.read()
Port = '/dev/ttyAMA0'
ser = serial.Serial(Port,115200,timeout=15)
while True:
send=s
ser.write(send.encode('utf-8'))
str1=ser.read(7).decode('utf-8')
if ('huanghe' in str(str1)):
print(str1+' '+str(i))
else:
print('get info is '+str(str1))
ser.close
注:uart连接一定要接地,否则会报错
开关机时间调整
#coding:utf-8
from machine import UART,Pin,RTC
import machine
import time,network
import ntptime
import os
led1=Pin(12,Pin.OUT)
led2=Pin(13,Pin.OUT)
rtc = RTC()
k=0
def linedetect():
uart=UART(1,baudrate=115200,tx=6,rx=7,timeout=1) #设置Pin6=tx,Pin7=rx
idsend='huanghe'
idreceive='changjiang'
uart.write(idsend)
time.sleep(5) #树莓派启动需要的时间,否则会反复重启
receive_data=uart.readline()
if (idreceive in str(receive_data)):
return 1
else:
return 0
def onoff(pinnum):
p = Pin(pinnum, Pin.OUT)
p.on()
time.sleep(0.5)
p.off()
def createconfigfile(filename):
uart=UART(1,baudrate=115200,tx=6,rx=7,timeout=1)
time.sleep(5)
readmsg = uart.readline()
if 'SSID' and 'PWD' and 'START' and 'END' and 'changjiang' in str(readmsg):
content = readmsg
with open(filename,mode='w',encoding='utf-8') as f:
f.write(content)
def readconfigfile(filename):
with open(filename,mode='r',encoding='utf-8') as f:
s=f.read().strip('\n').split(',')
if 'SSID' and 'PWD' and 'START' and 'END' and 'changjiang' in s:
ssid=s[0].split(':')[1]
pwd=s[1].split(':')[1]
startt=s[2].split(':')[1]
endt=s[3].split(':')[1]
return ssid,pwd,startt,endt
else:
print('config file format error,will drop it')
os.remove(filename)
# 同步时间
def sync_ntp():
ntptime.NTP_DELTA = 3155644800 # 可选 UTC+8偏移时间(秒),不设置就是UTC0
ntptime.host = 'ntp1.aliyun.com' # 可选,ntp服务器,默认是"pool.ntp.org"
try:
ntptime.settime() # 修改设备时间,到这就已经设置好了
except:
for i in range(6):
led1.value(1) #turn off 0是亮
time.sleep(0.1)
led1.value(0)
time.sleep(0.1)
print('同步失败')
# 联WIFI
def WIFI_Connect(ssid,pwd):
wlan = network.WLAN(network.STA_IF) #STA模式
wlan.active(True) #激活接口
start_time=time.time() #记录时间做超时判断
if not wlan.isconnected():
print('connecting to network...'+ssid)
wlan.connect(ssid, pwd) #输入WIFI账号密码
time.sleep(1)
while not wlan.isconnected():
if time.time()-start_time > 15 :
print('wifi Connected Timeout!')
os.remove('config.txt')
machine.reset()
if wlan.isconnected():
print('wifi was connected!')
try:
a,b,c,d=readconfigfile('config.txt')
except:
print('can not find config.txt,will create it!')
createconfigfile('config.txt')
time.sleep(2)
machine.reset()
WIFI_Connect(a,b)
print(a,b,c,d)
while True:
led2.value(1)
time.sleep(0.1)
led2.value(0)
time.sleep(0.1)
sync_ntp()
dt=time.localtime()
print(dt)
linestate=linedetect()
if linestate!=0:
print('on line')
if dt[3]>=int(d):
onoff(2)
else:
print('off line')
if (dt[3]<int(d) and dt[3]>=int(c)):
onoff(2)
自动同步上位机配置文件更新
ESP-32-C3程序
#coding:utf-8
from machine import UART,Pin,RTC
import machine
import time,network
import ntptime
import os
led1=Pin(12,Pin.OUT)
led2=Pin(13,Pin.OUT)
rtc = RTC()
k=0
def linedetect():
uart=UART(1,baudrate=115200,tx=6,rx=7,timeout=1) #设置Pin6=tx,Pin7=rx
idsend='huanghe'
idreceive='changjiang'
uart.write(idsend)
time.sleep(5) #树莓派启动需要的时间,否则会反复重启
receive_data=uart.readline()
if (idreceive in str(receive_data)):
return 1
else:
return 0
def onoff(pinnum):
p = Pin(pinnum, Pin.OUT)
p.on()
time.sleep(0.5)
p.off()
def createconfigfile(filename):
uart=UART(1,baudrate=115200,tx=6,rx=7,timeout=1)
time.sleep(5)
readmsg = uart.readline()
if 'SSID' and 'PWD' and 'START' and 'END' and 'changjiang' in str(readmsg):
content = readmsg
with open(filename,mode='w',encoding='utf-8') as f:
f.write(content)
def readconfigfile(filename):
with open(filename,mode='r',encoding='utf-8') as f:
s=f.read().strip('\n').split(',')
if 'SSID' and 'PWD' and 'START' and 'END' and 'changjiang' in s:
ssid=s[0].split(':')[1]
pwd=s[1].split(':')[1]
startt=s[2].split(':')[1]
endt=s[3].split(':')[1]
return ssid,pwd,startt,endt
else:
print('config file format error,will drop it')
os.remove(filename)
# 同步时间
def sync_ntp():
ntptime.NTP_DELTA = 3155644800 # 可选 UTC+8偏移时间(秒),不设置就是UTC0
ntptime.host = 'ntp1.aliyun.com' # 可选,ntp服务器,默认是"pool.ntp.org"
try:
ntptime.settime() # 修改设备时间,到这就已经设置好了
except:
for i in range(6):
led1.value(1) #turn off 0是亮
time.sleep(0.1)
led1.value(0)
time.sleep(0.1)
print('同步失败')
# 联WIFI
def WIFI_Connect(ssid,pwd):
wlan = network.WLAN(network.STA_IF) #STA模式
wlan.active(True) #激活接口
start_time=time.time() #记录时间做超时判断
if not wlan.isconnected():
print('connecting to network...'+ssid)
wlan.connect(ssid, pwd) #输入WIFI账号密码
time.sleep(1)
while not wlan.isconnected():
if time.time()-start_time > 15 :
print('wifi Connected Timeout!')
os.remove('config.txt')
machine.reset()
if wlan.isconnected():
print('wifi was connected!')
try:
a,b,c,d=readconfigfile('config.txt')
except:
print('can not find config.txt,will create it!')
createconfigfile('config.txt')
time.sleep(2)
machine.reset()
WIFI_Connect(a,b)
print(a,b,c,d)
while True:
led2.value(1)
time.sleep(0.1)
led2.value(0)
time.sleep(0.1)
sync_ntp()
dt=time.localtime()
print(dt)
linestate=linedetect()
if linestate!=0:
print('on line')
if dt[3]>=int(d):
onoff(2)
else:
print('off line')
if (dt[3]<int(d) and dt[3]>=int(c)):
onoff(2)
树莓派侧程序
#coding:utf-8
import serial
import time
with open('/home/pi/config.txt',mode='r',encoding='utf-8') as f:
s=f.read()
i=0
Port ="/dev/ttyAMA0"
ser = serial.Serial(Port,115200,timeout=1)
while True:
# send = 'SSID:HUAWEI-WULIAN,PWD:onlychina,START:6,END:20,changjiang'
send=s
i=i+1
ser.write(send.encode('utf-8'))
# str = ser.readline().decode()
str1 = ser.readline().decode('utf-8')
# time.sleep(5)
if('huanghe' in str(str1)):
print(str1+' '+str(i))
else:
print('can not get right info ,now info is '+str(str1))
with open('/home/pi/config.txt',mode='r',encoding='utf-8') as f:
s=f.read()
ser.close()
改进Ctrl+c的中断事件
#coding:utf-8
import serial
import time
try:
s=''
try:
with open('/home/pi/config.json',mode='r',encoding='utf-8') as f:
s=f.read()
except:
print("File 'config.json' can not find in dir /home/pi/,please create it first and run again.未能在/home/pi/目录下发现文件config.json,请按要求创建后再执行此程序")
i=0
Port ="/dev/ttyAMA0"
ser = serial.Serial(Port,115200,timeout=1)
while True:
# send = 'SSID:HUAWEI-WULIAN,PWD:onlychina,START:6,END:20,changjiang'
send=s
i=i+1
ser.write(send.encode('utf-8'))
# str = ser.readline().decode()
str1 = ser.readline().decode('utf-8')
# time.sleep(5)
if('hello' in str(str1)):
print(str1+' '+str(i))
else:
print('can not get right info ,now info is '+str(str1))
ser.close()
except KeyboardInterrupt:
print("Application exit!")
#coding:utf-8
from machine import UART,Pin,RTC
import machine
import time,network
import ntptime
import os
led1=Pin(12,Pin.OUT)
led2=Pin(13,Pin.OUT)
rtc = RTC()
k=0
def linedetect():
uart=UART(1,baudrate=115200,tx=6,rx=7,timeout=1) #设置Pin6=tx,Pin7=rx
idsend='hello'
idreceive='world'
uart.write(idsend)
time.sleep(5) #树莓派启动需要的时间,否则会反复重启
receive_data=uart.readline()
if (idreceive in str(receive_data)):
return 1
else:
return 0
def onoff(pinnum):
p = Pin(pinnum, Pin.OUT)
p.on()
time.sleep(0.5)
p.off()
def createconfigfile(filename):
uart=UART(1,baudrate=115200,tx=6,rx=7,timeout=1)
time.sleep(5)
readmsg = uart.readline()
if 'SSID' and 'PWD' and 'START' and 'END' and 'world' in str(readmsg):
content = readmsg
with open(filename,mode='w',encoding='utf-8') as f:
f.write(content)
def readconfigfile(filename):
with open(filename,mode='r',encoding='utf-8') as f:
s=f.read().strip('\n').split(',')
if 'SSID' and 'PWD' and 'START' and 'END' and 'world' in s:
ssid=s[0].split(':')[1]
pwd=s[1].split(':')[1]
startt=s[2].split(':')[1]
endt=s[3].split(':')[1]
return ssid,pwd,startt,endt
else:
print('config file format error,will drop it')
os.remove(filename)
# 同步时间
def sync_ntp():
ntptime.NTP_DELTA = 3155644800 # 可选 UTC+8偏移时间(秒),不设置就是UTC0
ntptime.host = 'ntp1.aliyun.com' # 可选,ntp服务器,默认是"pool.ntp.org"
try:
ntptime.settime() # 修改设备时间,到这就已经设置好了
except:
for i in range(6):
led1.value(1) #turn off 0是亮
time.sleep(0.1)
led1.value(0)
time.sleep(0.1)
print('同步失败')
# 联WIFI
def WIFI_Connect(ssid,pwd):
wlan = network.WLAN(network.STA_IF) #STA模式
wlan.active(True) #激活接口
start_time=time.time() #记录时间做超时判断
if not wlan.isconnected():
print('connecting to network...'+ssid)
wlan.connect(ssid, pwd) #输入WIFI账号密码
time.sleep(1)
while not wlan.isconnected():
if time.time()-start_time > 15 :
print('wifi Connected Timeout!')
os.remove('config.json')
machine.reset()
if wlan.isconnected():
print('wifi was connected!')
try:
a,b,c,d=readconfigfile('config.json')
except:
print('can not find config.json,will create it!')
createconfigfile('config.json')
time.sleep(2)
machine.reset()
WIFI_Connect(a,b)
print(a,b,c,d)
while True:
led2.value(1)
time.sleep(0.1)
led2.value(0)
time.sleep(0.1)
sync_ntp()
dt=time.localtime()
print(dt)
linestate=linedetect()
if linestate!=0:
print('on line')
if dt[3]>=int(d):
onoff(2)
else:
print('off line')
if (dt[3]<int(d) and dt[3]>=int(c)):
onoff(2)